Flutter custom dialog with radio button lists
- Programmer's Helpdesk
- May 3, 2020
- 1 min read
Updated: May 17, 2020
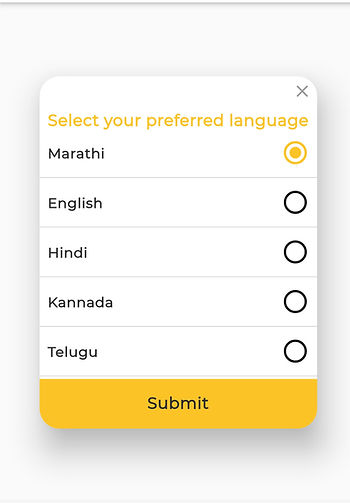
Create class for list item having text and radio button. I have created statelesswidget CustomRow and CustomRowModel.
class CustomRowModel {
bool selected;
String title;
CustomRowModel({this.selected, this.title});
}
class CustomRow extends StatelessWidget {
final CustomRowModel model;
CustomRow(this.model);
@override
Widget build(BuildContext context) {
return Padding(
padding:
const EdgeInsets.only(left: 8.0, right: 8.0, top: 3.0, bottom: 3.0),
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
// I have used my own CustomText class to customise TextWidget.
CustomText(
label: model.title,
),
this.model.selected
? Icon(
Icons.radio_button_checked,
color: Colors.amber,
)
: Icon(Icons.radio_button_unchecked),
],
),
);
}
}
Now create dialog design. In my dialog, having cancel icon, heading, custom listview and button 'Submit'.
dialogContent(BuildContext context) {
return Container(
child: Column(
children: <Widget>[
Container(
margin: EdgeInsets.all(5.0),
alignment: Alignment.topRight,
child: Icon(
Icons.close,
color: Colors.grey,
size: 20.0,
),
),
Container(
margin: EdgeInsets.only(top: 5.0, bottom: 5.0),
color: Colors.white,
child: CustomText(
fontSize: 16.0,
label: "Select your preferred language",
labelColor: AppColors.dialogTitleColor,
fontWeight: FontWeight.bold,
),
),
Flexible(
child: new MyDialogContent(),//Custom ListView
),
SizedBox(
height: 50,
width: double.infinity,
child: FlatButton(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.only(
bottomLeft: Radius.circular(20.0),
bottomRight: Radius.circular(20.0),
),
),
onPressed: () {},
color: AppColors.dialogTitleColor,
textColor: Colors.black,
child: CustomText(
label: "Submit",
fontWeight: FontWeight.bold,
fontSize: 16.0,
),
),
),
],
),
);
}
Now to Create LanguageSelection statefulwidget to show our customised dialog.
class LanguageSelection extends StatefulWidget {
@override
State<StatefulWidget> createState() {
return LanguageSelectionState();
}
}
class LanguageSelectionState extends State<LanguageSelection> {
@override
void initState() {
super.initState();
}
@override
Widget build(BuildContext context) {
return Container(
margin: EdgeInsets.only(top: 50.0, bottom: 50.0),
alignment: Alignment.center,
child: Dialog(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.all(
Radius.circular(20.0),
),
),
elevation: 25.0,
backgroundColor: Colors.white,
child: dialogContent(context),
),
);
}
}
MyDialogContent statefulwidget I have create list item and handle state of after clicking on radio button to show selected/deselected.
class MyDialogContent extends StatefulWidget {
@override
_MyDialogContentState createState() => new _MyDialogContentState();
}
class _MyDialogContentState extends State<MyDialogContent> {
List<CustomRowModel> sampleData = new List<CustomRowModel>();
@override
void initState() {
super.initState();
sampleData.add(CustomRowModel(title: "Marathi", selected: false));
sampleData.add(CustomRowModel(title: "English", selected: false));
sampleData.add(CustomRowModel(title: "Hindi", selected: false));
sampleData.add(CustomRowModel(title: "Kannada", selected: false));
sampleData.add(CustomRowModel(title: "Telugu", selected: false));
sampleData.add(CustomRowModel(title: "Gujarathi", selected: false);
sampleData.add(CustomRowModel(title: "Rajsthani", selected: false);
sampleData.add(CustomRowModel(title: "Punjabi", selected: false));
}
@override
Widget build(BuildContext context) {
return sampleData.length == 0
? Container()
: Container(
child: ListView.separated(
separatorBuilder: (context, index) => Divider(
color: Colors.grey,
),
scrollDirection: Axis.vertical,
shrinkWrap: true,
itemCount: sampleData.length,
itemBuilder: (BuildContext context, int index) {
return new InkWell(
//highlightColor: Colors.red,
//splashColor: Colors.blueAccent,
onTap: () {
setState(() {
sampleData.forEach((element) => element.selected = false);
sampleData[index].selected = true;
});
},
child: new CustomRow(sampleData[index]),
);
},
),
);
}
}
Comentários